Write a C program to
implement sorting of an array of elements.
SOURCE CODE:
/*Program to implement sorting of an array of elements.*/
#include<stdio.h>
#include<conio.h>
void main()
{
int a[30],n,i,j,temp;
clrscr();
printf("\nEnter the array size:");
scanf("%d",&n);
printf("\nEnter %d elements:\n",n);
for(i=0;i<n;i++)
scanf("%d",&a[i]);
printf("\nBefore sorting array is:\n");
for(i=0;i<n;i++)
printf("%d\t",a[i]);
printf("\nAfter sorting array is:\n");
for(i=0;i<n;i++)
{
for(j=i+1;j<n;j++)
{
if(a[i]>a[j])
{
temp=a[i];
a[i]=a[j];
a[j]=temp;
}
}
}
for(i=0;i<n;i++)
printf("%d\t",a[i]);
}
OUTPUT:
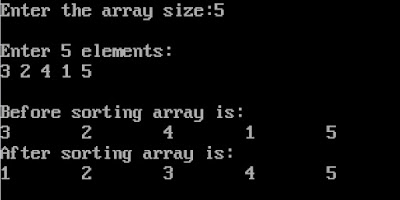
Write a C program to input two m x n matrices, check the compatibility and perform addition them.
SOURCE CODE:
/*Program to perform sum of two matrices*/
#include<stdio.h>
#include<conio.h>
void main()
{
int a[20][20],b[20][20],c[20][20],i,j,r1,c1,r2,c2;
clrscr();
printf("Enter first matrix row and column size:");
scanf("%d%d",&r1,&c1);
printf("Enter second matrix row and column size:");
scanf("%d%d",&r2,&c2);
if((r1!=r2)||(c1!=c2))
printf("\nMatrix addition is not possible.");
else
{
printf("\nEnter first matrix elements %d X %d:\n",r1,c1);
for(i=0;i<r1;i++)
for(j=0;j<c1;j++)
scanf("%d",&a[i][j]);
printf("\nEnter second matrix elements %d X %d:\n",r2,c2);
for(i=0;i<r2;i++)
for(j=0;j<c2;j++)
scanf("%d",&b[i][j]);
for(i=0;i<r1;i++)
{
for(j=0;j<c1;j++)
{
c[i][j]=a[i][j]+b[i][j];
}
}
printf("\n::Addition of Two Matrices is::\n");
for(i=0;i<r1;i++)
{
for(j=0;j<c1;j++)
{
printf("%d\t",c[i][j]);
}
printf("\n");
}
}
}
OUTPUT:
Write a C program to input two m x n matrices, check the compatibility and perform multiplication them.
SOURCE CODE:
/*Program to perform product of two matrices*/
#include<stdio.h>
#include<conio.h>
void main()
{
int a[20][20],b[20][20],c[20][20],i,j,k,r1,c1,r2,c2;
clrscr();
printf("Enter first matrix row and column size:");
scanf("%d%d",&r1,&c1);
printf("Enter second matrix row and column size:");
scanf("%d%d",&r2,&c2);
if(c1!=r2)
printf("\nMatrix multiplication is not possible.");
else
{
printf("\nEnter first matrix elements %d X %d:\n",r1,c1);
for(i=0;i<r1;i++)
for(j=0;j<c1;j++)
scanf("%d",&a[i][j]);
printf("\nEnter second matrix elements %d X %d:\n",r2,c2);
for(i=0;i<r2;i++)
for(j=0;j<c2;j++)
scanf("%d",&b[i][j]);
for(i=0;i<r1;i++)
{
for(j=0;j<c2;j++)
{
c[i][j]=0;
for(k=0;k<c1;k++)
{
c[i][j]=c[i][j]+a[i][k]*b[k][j];
}
}
}
printf("\n::Multiplication of Two Matrices is::\n");
for(i=0;i<r1;i++)
{
for(j=0;j<c2;j++)
{
printf("%d\t",c[i][j]);
}
printf("\n");
}
}
}
OUTPUT:
No comments:
Post a Comment